Read Multiple Analog values with esp8266/Nodemcu
- eMbed Workshop
- Apr 10, 2023
- 1 min read
Updated: Jun 9, 2023
We will demonstrate in this tutorial how to read multiple analog sensor values from a single analog input pin. Instead of having multiple analog pins like other boards, the esp8266 only has one analog pin. We make it possible to read multiple analog values using just one pin.
Needed equipment:
esp8266/nodemcu
analog sensors
diodes
breadboard
jumper wires
BASICS
By switching sensors on and off, similar to multiplexing, we achieve this. In the first stage, we turn on one sensor, read the analog data from that sensor, then turn on the next sensor, switch off the first sensor, then receive data from the second sensor that was just turned on.
Wiring Diagram
Vcc of sensor1 -> d1 (5)
Vcc of sensor2 -> d2 (4)
grounds of sensors -> grounds
analog output of sensors -> diodes positive(+) side
diodes negative(-) side -> A0 of esp8266
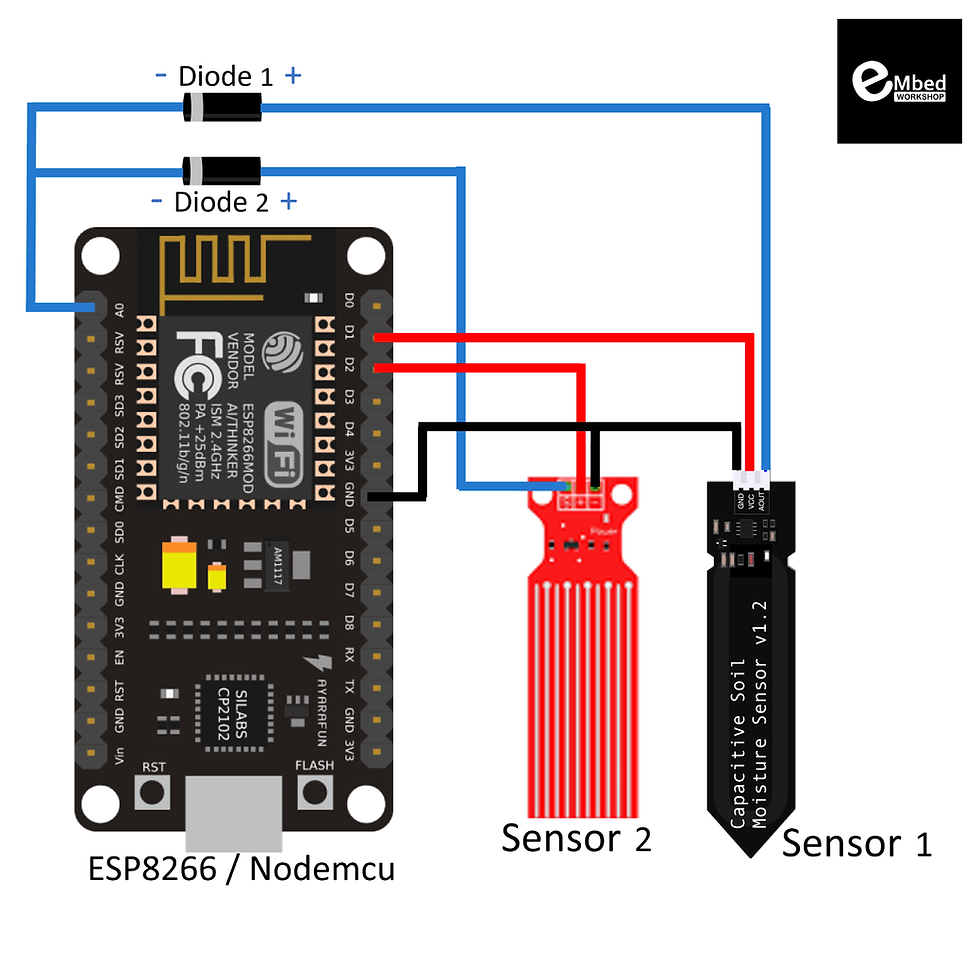
CODE
int val1 = 0;
int val2 = 0;
const int sensor1_pin = 5;
const int sensor2_pin = 4;
void setup() {
Serial.begin(9600);
pinMode(sensor1_pin, OUTPUT);
pinMode(sensor2_pin, OUTPUT);
}
int sensorRead1() {
digitalWrite(sensor1_pin, HIGH); // sensor1 turn On
digitalWrite(sensor2_pin, LOW); // sensor2 turn Off
return analogRead(A0);
}
int sensorRead2() {
digitalWrite(sensor1_pin, LOW); // sensor1 turn Off
digitalWrite(sensor2_pin, HIGH); // sensor2 turn on
return analogRead(A0);
}
void loop() {
val1 = sensorRead1(); // Read Analog value of first sensor
delay(200);
val2 = sensorRead2(); // Read Analog value of second sensor
delay(200);
Serial.println("sensor 1 reading = ");
Serial.print(val1);
Serial.print(", sensor 2 reading = ");
Serial.println(val2);
}
Comments